Saturday, September 8, 2012
Saturday, September 1, 2012
GridView with DataSet ,DataTable, DataReader
DataBase Design
Default.aspx
Default.aspx.cs
protected void Button1_Click(object sender, EventArgs e)
{
/* dataset */
con = new SqlConnection("user id=sa;password=uday;database=uday;data source=localhost");
cmd=new SqlCommand("select *from customers", con);
con.Open();
da=new SqlDataAdapter(cmd);
ds = new DataSet();
da.Fill(ds,"customers");
GridView1.DataMember = "customers";
GridView1.DataSource = ds;
GridView1.DataBind();
con.Close();
}
protected void Button3_Click(object sender, EventArgs e)
{
/* DataTable */
con = new SqlConnection("user id=sa;password=uday;database=uday;data source=localhost");
cmd = new SqlCommand("select *from customers", con);
con.Open();
da = new SqlDataAdapter(cmd);
dt = new DataTable();
da.Fill(dt);
GridView1.DataSource = dt;
GridView1.DataBind();
con.Close();
}
protected void Button2_Click1(object sender, EventArgs e)
{
/* datareader */
con = new SqlConnection("user id=sa;password=uday;database=uday;data source=localhost");
cmd = new SqlCommand("select *from customers", con);
con.Open();
SqlDataReader dr;
dr = cmd.ExecuteReader();
GridView1.DataSource = dr;
GridView1.DataBind();
con.Close();
}
Output:
Default.aspx
Default.aspx.cs
protected void Button1_Click(object sender, EventArgs e)
{
/* dataset */
con = new SqlConnection("user id=sa;password=uday;database=uday;data source=localhost");
cmd=new SqlCommand("select *from customers", con);
con.Open();
da=new SqlDataAdapter(cmd);
ds = new DataSet();
da.Fill(ds,"customers");
GridView1.DataMember = "customers";
GridView1.DataSource = ds;
GridView1.DataBind();
con.Close();
}
protected void Button3_Click(object sender, EventArgs e)
{
/* DataTable */
con = new SqlConnection("user id=sa;password=uday;database=uday;data source=localhost");
cmd = new SqlCommand("select *from customers", con);
con.Open();
da = new SqlDataAdapter(cmd);
dt = new DataTable();
da.Fill(dt);
GridView1.DataSource = dt;
GridView1.DataBind();
con.Close();
}
protected void Button2_Click1(object sender, EventArgs e)
{
/* datareader */
con = new SqlConnection("user id=sa;password=uday;database=uday;data source=localhost");
cmd = new SqlCommand("select *from customers", con);
con.Open();
SqlDataReader dr;
dr = cmd.ExecuteReader();
GridView1.DataSource = dr;
GridView1.DataBind();
con.Close();
}
Output:
Friday, August 31, 2012
Access Specifiers in C#.
They are used to define scope of the Class or its Members i,e who can access it and who cannot Access it.
Here we will discuss about each access specifier with an example.
.Net Supports five Access Specifiers.
1 Public.
2 Private.
3 Protected.
4 Internal.
5 Protected Internal.
public:
So any member declared as public can be accessed from the outside the class.
We can access from other class using object reference.
Example
File-New- Project- Console Application.
In the above example we have created a object in Derived Class , since the variable is public it can be accessed from outside the class.
output
private
Members declared as private are not Accessible outside the class.
A class under Namespace cannot be declared as private.
Example
Output:
Example for Public and Private Access Specifier.
output
2 Example
output
Protected
If the members are declared as protected , then they can be accessed only From the child class., it is same as private.
Note: A class under Namespace cannot be declared as private.
This access specifier is used when we need to use Inheritance in the program.
output
Internal
Members are declared as internal under a class than they r accessible only with in the Project either from the Child Class or a Non Child Class. These are accessible only within the Assembly.
internal is the default Access Specifier for the Class.
output
Protected Internal
Members declared as ProtectedInternal under a Class enjoys Dual Scope. i,e with the project they behave as internal members and provide access to all the classes, outside the project they change to Protected and still provide access to there child classes.
Here we will discuss about each access specifier with an example.
.Net Supports five Access Specifiers.
1 Public.
2 Private.
3 Protected.
4 Internal.
5 Protected Internal.
public:
So any member declared as public can be accessed from the outside the class.
We can access from other class using object reference.
Example
File-New- Project- Console Application.
In the above example we have created a object in Derived Class , since the variable is public it can be accessed from outside the class.
output
private
Members declared as private are not Accessible outside the class.
A class under Namespace cannot be declared as private.
Example
Output:
Example for Public and Private Access Specifier.
output
2 Example
output
Protected
If the members are declared as protected , then they can be accessed only From the child class., it is same as private.
Note: A class under Namespace cannot be declared as private.
This access specifier is used when we need to use Inheritance in the program.
output
Another Example of working with Protected Access Specifier.
Internal
Members are declared as internal under a class than they r accessible only with in the Project either from the Child Class or a Non Child Class. These are accessible only within the Assembly.
internal is the default Access Specifier for the Class.
output
Protected Internal
Members declared as ProtectedInternal under a Class enjoys Dual Scope. i,e with the project they behave as internal members and provide access to all the classes, outside the project they change to Protected and still provide access to there child classes.
Output:
Add a new project under the solution of type Console Application, name it as ConsoleApplication2 & rename the default file Program.cs as Four.cs so that class name also changes as Four, now write the following code in it by adding the reference of ConsoleApplication1 assembly from its physical location.
Sunday, August 26, 2012
ICALL BACK EVENT HANDLER
We have to play with 4 functions,
Two client side functions i.e. java script function,
JavaScript Functions
RaiseCallbackEvent function gets call automatically whenever there is a CallbackEvent. And after this function 2nd function i.e. GetCallbackResult get called automatically.
DataBase Design
<html xmlns="http://www.w3.org/1999/xhtml">
function ReceiveServerData(retValue) {
using System.Data.SqlClient;
public partial class AddPage : System.Web.UI.Page,ICallbackEventHandler
}
public void RaiseCallbackEvent(String eventArgument)
Output
Two server side function i.e. c# (in this case).
1 function ReceiveServerData(retValue)
2 function Checkusername
Two client side functions i.e. java script function,
JavaScript Functions
RaiseCallbackEvent function gets call automatically whenever there is a CallbackEvent. And after this function 2nd function i.e. GetCallbackResult get called automatically.
DataBase Design
<html xmlns="http://www.w3.org/1999/xhtml">
function ReceiveServerData(retValue) {
using System.Data.SqlClient;
public partial class AddPage : System.Web.UI.Page,ICallbackEventHandler
}
public void RaiseCallbackEvent(String eventArgument)
Output
Two server side function i.e. c# (in this case).
1 function ReceiveServerData(retValue)
2 function Checkusername
.cs functions
1 public void RaiseCallbackEvent(String eventArgument)
2 public String GetCallbackResult()
AddPage.aspx
<head runat="server">
<title></title>
<script type="text/javascript" language="javascript">
function Checkusername ()
{
var values = document.getElementById("txtEname").value;
CallServer(values, "");
}
if (retValue == "N") {
alert("Please select another name; empname already exists");
document.getElementById("txtEname").value = "";
document.getElementById("txtEname").focus();
}
}
</script>
</head>
<body>
<form id="form1" runat="server">
<div>
<table>
<tr>
<td>
<asp:Label ID="Label1" runat="server" Text="Eno"></asp:Label>
</td>
<td>
<asp:TextBox ID="txtEno" runat="server" ></asp:TextBox>
</td>
</tr>
<tr>
<td>
<asp:Label ID="Label2" runat="server" Text="Ename"></asp:Label>
</td>
<td>
<asp:TextBox ID="txtEname" runat="server" onblur="javascript:Checkusername()"></asp:TextBox>
</td>
</tr>
<tr>
<td>
<asp:Label ID="Label3" runat="server" Text="Designation"></asp:Label>
</td>
<td>
<asp:TextBox ID="txtDesignation" runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td colspan="2" align="center">
<asp:Button ID="btnSave" runat="server" Text="Save" onclick="btnSave_Click" />
</td>
</tr>
</table>
</div>
</form>
</body>
</html>
AddPage.aspx.cs
using System.Configuration;
{
protected String returnValue;
protected void Page_Load(object sender, EventArgs e)
{
String cbReference = Page.ClientScript.GetCallbackEventReference(this, "arg","ReceiveServerData", "context");
string callbackScript = "function CallServer(arg,context)" + "{" + cbReference + ";}";
Page.ClientScript.RegisterClientScriptBlock(this.GetType(), "CallServer", callbackScript,true);
}
protected void btnSave_Click(object sender, EventArgs e)
{
SqlConnection con = new SqlConnection("user id=sa;password=uday;database=uday;data source=localhost");
con.Open();
SqlCommand cmd = new SqlCommand("insert into employee values(" + txtEno.Text + ",'" + txtEname.Text + "','" + txtDesignation.Text + "')", con);
int i=cmd.ExecuteNonQuery();
if(i>0)
{
Response.Redirect("Default.aspx");
}
con.Close();
{
SqlConnection con = new SqlConnection("user id=sa;password=uday;database=uday;data source=localhost");
SqlCommand com = new SqlCommand("select *from employee where ename='" +eventArgument + "'", con);
con.Open();
SqlDataReader dr;
dr = com.ExecuteReader();
if (dr.HasRows == true)
{
returnValue = "N";
}
else
{
returnValue = "Y";
}
con.Close();
}
public String GetCallbackResult()
{
return returnValue;
}
}
<head runat="server">
<title></title>
<script type="text/javascript" language="javascript">
function Checkusername ()
{
var values = document.getElementById("txtEname").value;
CallServer(values, "");
}
if (retValue == "N") {
alert("Please select another name; empname already exists");
document.getElementById("txtEname").value = "";
document.getElementById("txtEname").focus();
}
}
</script>
</head>
<body>
<form id="form1" runat="server">
<div>
<table>
<tr>
<td>
<asp:Label ID="Label1" runat="server" Text="Eno"></asp:Label>
</td>
<td>
<asp:TextBox ID="txtEno" runat="server" ></asp:TextBox>
</td>
</tr>
<tr>
<td>
<asp:Label ID="Label2" runat="server" Text="Ename"></asp:Label>
</td>
<td>
<asp:TextBox ID="txtEname" runat="server" onblur="javascript:Checkusername()"></asp:TextBox>
</td>
</tr>
<tr>
<td>
<asp:Label ID="Label3" runat="server" Text="Designation"></asp:Label>
</td>
<td>
<asp:TextBox ID="txtDesignation" runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td colspan="2" align="center">
<asp:Button ID="btnSave" runat="server" Text="Save" onclick="btnSave_Click" />
</td>
</tr>
</table>
</div>
</form>
</body>
</html>
AddPage.aspx.cs
using System.Configuration;
{
protected String returnValue;
protected void Page_Load(object sender, EventArgs e)
{
String cbReference = Page.ClientScript.GetCallbackEventReference(this, "arg","ReceiveServerData", "context");
string callbackScript = "function CallServer(arg,context)" + "{" + cbReference + ";}";
Page.ClientScript.RegisterClientScriptBlock(this.GetType(), "CallServer", callbackScript,true);
}
protected void btnSave_Click(object sender, EventArgs e)
{
SqlConnection con = new SqlConnection("user id=sa;password=uday;database=uday;data source=localhost");
con.Open();
SqlCommand cmd = new SqlCommand("insert into employee values(" + txtEno.Text + ",'" + txtEname.Text + "','" + txtDesignation.Text + "')", con);
int i=cmd.ExecuteNonQuery();
if(i>0)
{
Response.Redirect("Default.aspx");
}
con.Close();
{
SqlConnection con = new SqlConnection("user id=sa;password=uday;database=uday;data source=localhost");
SqlCommand com = new SqlCommand("select *from employee where ename='" +eventArgument + "'", con);
con.Open();
SqlDataReader dr;
dr = com.ExecuteReader();
if (dr.HasRows == true)
{
returnValue = "N";
}
else
{
returnValue = "Y";
}
con.Close();
}
public String GetCallbackResult()
{
return returnValue;
}
}
Monday, August 20, 2012
Working with JSON
JSON (JavaScript Object Notation) is one lightweight data exchange format.
You don’t need to download an additional library to serialize/deserialize your objects to/from JSON. Since .NET 3.5, .NET can do it natively.
Add a reference to your project to “System.Web.Extensions.dll”
File->New->Website
Add a Class(Static.cs)
Now write the Following Code in Static.cs
using System;
/// <summary>
public String Technologies()
public ArrayList EmpDetails()
public string JsonString()
Now Add New Item
Default.aspx
<html xmlns="http://www.w3.org/1999/xhtml">
Default.aspx.cs
using System.Web.UI;
public partial class Default3 : System.Web.UI.Page
Now Run
Paste the following output and Click on Viewer
using System.Web.UI;
public partial class Default3 : System.Web.UI.Page
using System;
/// <summary>
public String Technologies()
public ArrayList EmpDetails()
public string JsonString()
Now Add New Item
Default.aspx
<html xmlns="http://www.w3.org/1999/xhtml">
Default.aspx.cs
using System.Web.UI;
public partial class Default3 : System.Web.UI.Page
Now Run
Paste the following output and Click on Viewer
using System.Web.UI;
public partial class Default3 : System.Web.UI.Page
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Script.Serialization;
using System.Collections;
/// Summary description for Static
/// </summary>
public class Static
{
JavaScriptSerializer jss = new JavaScriptSerializer();
{
string jsonString = string.Empty;
string[] courses = { "asp.net", "sqlserver", "silverlight", "sharepoint", "iPhone", "vb.net", "java", "php" };
Dictionary<string, string[]> dicEmpInfo = new Dictionary<string, string[]>();
dicEmpInfo.Add("Technologies", courses);
jsonString = jss.Serialize(dicEmpInfo);
return jsonString;
}
{
Dictionary<string, string> dicpersonalinfo = new Dictionary<string, string>();
dicpersonalinfo.Add("First Name", "Uday");
dicpersonalinfo.Add("LastName", "Kumar");
Dictionary<string, string> dicpersonalInfo2 = new Dictionary<string, string>();
dicpersonalInfo2.Add("FirstName", "Anvesh");
dicpersonalInfo2.Add("LastName", "G");
Dictionary<string, string> dicpersonalInfo3 = new Dictionary<string, string>();
dicpersonalInfo3.Add("FirstName", "Sunitha");
dicpersonalInfo3.Add("LastName", "Kumari");
Dictionary<string, string> dicpersonalInfo4 = new Dictionary<string, string>();
dicpersonalInfo4.Add("FirstName", "Badri");
dicpersonalInfo4.Add("LastName", "Nath");
ArrayList empDetails = new ArrayList();
empDetails.Add(dicpersonalinfo);
empDetails.Add(dicpersonalInfo2);
empDetails.Add(dicpersonalInfo3);
empDetails.Add(dicpersonalInfo4);
//string strJson = jss.Serialize(empDetails);
return empDetails;
}
{
string jsonString1 = string.Empty;
ArrayList arr = EmpDetails();
jsonString1 = jss.Serialize(arr);
return jsonString1;
}
}
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
</div>
</form>
</body>
</html>
using System.Web.UI.WebControls;
{
protected void Page_Load(object sender, EventArgs e)
{
Static obj = new Static();
string str = obj.Technologies();
Response.Write(str);
}
}
Now Go to
For Second Method in static.cs
Default.aspx.cs
using System.Web.UI.WebControls;
{
protected void Page_Load(object sender, EventArgs e)
{
Static obj = new Static();
string str = obj.Technologies();
// Response.Write(str);
string str1 = obj.JsonString();
Response.Write(str1);
}
}
copy the output and
Now Go to
Subscribe to:
Posts (Atom)
Python -3
Lists It is used to store Collection of data. Lists are created using square brackets: List Items Order cannot be changed. It can have dup...
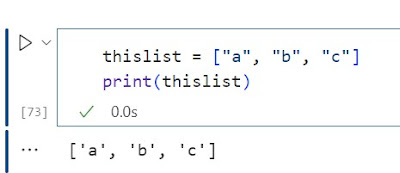
-
Visual Studio 2015 installation will not have Crystal Reports and hence we need to install it you can download it from the following locat...
-
SOLID principles allows object oriented programming implementation in an efficient better way into project development S-->Single R...
-
Step 1: Download and Install Latest Node.js URL : https://nodejs.org/en/download/ Step 2: Install Visual Studio Code (URL: https:/...