Wednesday, September 6, 2023
Jquery MultiSelect DropDown
Friday, February 11, 2022
TypeScript Part 4
Static Member
The term static refers to memory.
The static memory once allocated for an object will be used for all other objects.
It occupies more memory
The static members are declared by using the keyword static and they are accessible by using class name.
Non Static Member
It uses dynamic memory.
The dynamic memory is allocated for every object individually. Hence it is more secure, and occupies less memory.
The non static members are accessible with in the class by using "this" keyword.
Example
Output
s=1
n=0
s=2
n=0
s=3
n=0
Static Keyword /Access Specifier
-------------------------------
*static keyword can be applied to a field (or) method
1.static field
2.static method
static field:
-------------
*the field attached to a class is called "static field"
*static field will be allocated with memory only single time, it will be
shared by all the objects of a class
*static field can be referenced only with class name, not with object variable
static method:
--------------
*the method attached to a class is called "static method"
*static method can access only static fields
*static method can be referenced only with class name, not with object variable
Tuesday, February 8, 2022
TypeScript Part 3
Before going into OOPS, we need to know about the Object based programming system
Object Based Programming System
Features: Supports code reusability and code separation
Support Dynamic Memory Allocation
Can be Integrated with other programming system
Issues:
It will not support Inheritance
It will not support dynamic polymorphism
Code Extensibiity Issues
Ex: Javascript , VB Script
Object Oriented Programming
Features
Code Reusability
Code Separation
Dynamic Memory Allocation
Code Extensibility
Issues
Uses more memory
Slow than other programming system
Complex in configuration
OOPS IN TypeScript
Object oriented programming is provided with 4 Synopsis
1 Data Encapsulation
Combing/Binding related fields and methods as one unit is Called as "Data Encapsulation"
Practical:
Class is an implementation of data Encapsulation.
2 Data Abstraction
Hiding secured Data and functionality from user with the help of access specifiers is Called "Data Abstraction"
Practical:
Object is an implementation of data Abstraction.
TypeScript part 2
Variables in TypeScript
Variables are named storage locations in memory where you can store a value and use it as part of any expression.
TypeScript is in strict Mode of JavaScript and hence declaring variables in mandatory.
Variables in typescript can be declared by using the following reasons.
a) Var
b) let
c) const
var is used to define function scope variables so that it is accessible from any location within the function.
let is used to define block scope variable and hence it cannot be accessed outside the block.
const is used to define block scope variables which must be initialized with a value and cannot be shadowed.
shadowing is the process of using the same name identifier with different value within the scope.
Syntax
Sunday, February 6, 2022
Python -3
Lists It is used to store Collection of data. Lists are created using square brackets: List Items Order cannot be changed. It can have dup...
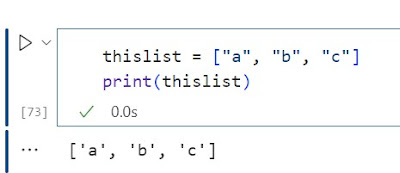
-
Visual Studio 2015 installation will not have Crystal Reports and hence we need to install it you can download it from the following locat...
-
SOLID principles allows object oriented programming implementation in an efficient better way into project development S-->Single R...
-
Step 1: Download and Install Latest Node.js URL : https://nodejs.org/en/download/ Step 2: Install Visual Studio Code (URL: https:/...