Note: Angular will not allow multiple directive in single elements.
Tuesday, September 26, 2023
Working with Angular Condition with Multiple Directives
Working with Angular Condition With UI Example
Working with Angular Condition with UI Example
Angular can handle conditions in UI using typescript that is using selection statements such as if else, switch and case.
Conditions can be handle by using angular directive such as "ngIf"
ngIf uses a boolean value to add or remove any DOM element dynamically.
Syntax
<div *ngIf =true/false > </div>
Example
Add a new component
ng g c angularconditionsui
.ts file changes
ScreenShot
.html Changes
Class Binding in Angular
Example Iteration for even and Odd Properties
Apply a CSS Class to a table row over iteration.
Syntax:
<tr class="className>
[class.ClassName]=true/false
Add a new Component
ng g c datatable
Goto datatablecomponent.ts and write the below code
Binding Form Data in angular
The data defined in the form element can be accessed and used in controller by using various techniques.
1 Interpolation 2 Two way binding
In Interpolation technique , the reference for form element is defined by using "#" and a value property is used to refer the element value
Syntax:
<input type="text" #uname> { {uname.value }}
Dealing with Forms
Example: Accessing Index over iterations
Add a new Component
ng g c datatable
Goto datatablecomponent.ts and write the below code
Output
Angular Iteration Properties with Example
The directive *ng For uses a iterator pattern to repeat any element and read the values from collection in a sequential order.
Angular iterations are provided with a set of properties that can access the iteration counter details which include the following
index--> It is a number Type
It returns the index number of iterator in an iteration.
It is a zero index based.
first--> It is boolean Type
It returns true if iteration counter is the first iterator
last--> It is boolean Type
It returns true if iteration counter is the last iterator
even--> It is boolean Type
It returns true for even occurances in iteration.
odd--> It is boolean Type
It returns true for odd occurances in iteration
trackBy -->It is a string(function)
It uses a function to track the changes in iteration.
Example
.ts File changes
.html File Changes
appmodule.ts
Output:

On Clicking on update products
Python -3
Lists It is used to store Collection of data. Lists are created using square brackets: List Items Order cannot be changed. It can have dup...
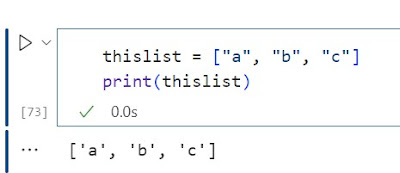
-
Visual Studio 2015 installation will not have Crystal Reports and hence we need to install it you can download it from the following locat...
-
SOLID principles allows object oriented programming implementation in an efficient better way into project development S-->Single R...
-
Step 1: Download and Install Latest Node.js URL : https://nodejs.org/en/download/ Step 2: Install Visual Studio Code (URL: https:/...