File->New->Project
Click on Ok Button
Design the database as shown below:
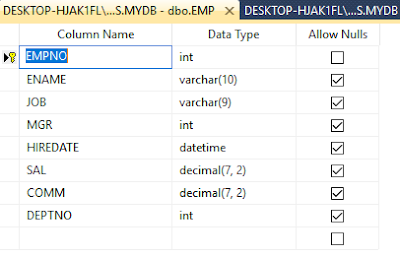
Now, Add a new ADO.NET Data Model, From the Model Folder
Click on Add
Click on Next
Click on New Connection
Click on Test Connection. Once the test connection is success
Click on Ok Button
Click on Next Button
Click on Next Button, Select the table Name on which you want to do CRUD Operations
Click on Finish Button
Now, we need to Add Angular Js Plugin
Click on Install Button
Once Installed you can see in the script folder
Now, we will Add a EmployeeController and Add the below code
EmployeeController:
public class EmployeeController : Controller
{
// GET: Employee
public ActionResult Index()
{
return View();
}
/// <summary>
/// This method is used to Get the Details of the Employee
/// </summary>
/// <returns>JSONRESULT</returns>
public JsonResult GetEmployeeDetails()
{
using (MYDBEntities Obj = new MYDBEntities())
{
List<EMP> Emp = Obj.EMPs.ToList();
return Json(Emp, JsonRequestBehavior.AllowGet);
}
}
/// <summary>
/// Insert an Employee
/// </summary>
/// <param name="Employe">emp</param>
/// <returns>String</returns>
[HttpPost]
public string InsertEmployee(EMP Employe)
{
if (Employe != null)
{
using (MYDBEntities Obj = new MYDBEntities())
{
Obj.EMPs.Add(Employe);
Obj.SaveChanges();
return "Employee Added Successfully";
}
}
else
{
return "Employee Not Inserted! Try Again";
}
}
/// <summary>
/// This method is used to Delete an Employee
/// </summary>
/// <param name="Employe">emp</param>
/// <returns>String</returns>
public string DeleteEmployee(EMP emp)
{
if (emp != null)
{
using (MYDBEntities Obj = new MYDBEntities())
{
var Emp_ = Obj.Entry(emp);
if (Emp_.State == System.Data.Entity.EntityState.Detached)
{
Obj.EMPs.Attach(emp);
Obj.EMPs.Remove(emp);
}
Obj.SaveChanges();
return "Employee Deleted Successfully";
}
}
else
{
return "Employee Not Deleted! Try Again";
}
}
public string UpdateEmployee(EMP Employe)
{
if (Employe != null)
{
using (MYDBEntities Obj = new MYDBEntities())
{
var Emp_ = Obj.Entry(Employe);
EMP EmpObj = Obj.EMPs.Where(x => x.EMPNO == Employe.EMPNO).FirstOrDefault();
EmpObj.ENAME = Employe.ENAME;
EmpObj.JOB = Employe.JOB;
EmpObj.DEPTNO = Employe.DEPTNO;
EmpObj.MGR = Employe.MGR;
EmpObj.SAL = Employe.SAL;
Obj.SaveChanges();
return "Employee Updated Successfully";
}
}
else
{
return "Employee Not Updated! Try Again";
}
}
}
Javascript Code:
/// <reference path="angular.js" />
var app = angular.module("myApp", []);
app.controller("myController", function ($scope, $http) {
$scope.InsertData = function () {
var Action = document.getElementById("btnSave").getAttribute("value");
if (Action == "Submit") {
$scope.Employe = {};
$scope.Employe.EMPNO = $scope.EmpNumber;
$scope.Employe.ENAME = $scope.EmpName;
$scope.Employe.JOB = $scope.EmpJob;
$scope.Employe.MGR = $scope.EmpMgrId;
$scope.Employe.SAL = $scope.EmpSalary;
$scope.Employe.DEPTNO = $scope.EmpDept;
$http({
method: "post",
url: "http://localhost:61289/Employee/InsertEmployee",
datatype: 'json',
data: JSON.stringify($scope.Employe)
}).then(function (response) {
alert(response.data);
$scope.Employe.EMPNO = "";
$scope.Employe.ENAME = "";
$scope.Employe.JOB = "";
$scope.Employe.MGR = "";
$scope.Employe.SAL = "";
$scope.Employe.DEPTNO = "";
$scope.GetAllData();
})
} else {
debugger;
$scope.Employe = {};
$scope.Employe.ENAME = $scope.EmpName;
$scope.Employe.JOB = $scope.EmpJob;
$scope.Employe.MGR = $scope.EmpMgrId;
$scope.Employe.SAL = $scope.EmpSalary;
$scope.Employe.DEPTNO = $scope.EmpDept;
$scope.Employe.EMPNO = document.getElementById("EmpNumber_").value;
$http({
method: "post",
url: "http://localhost:61289/Employee/UpdateEmployee",
datatype: "json",
data: JSON.stringify($scope.Employe)
}).then(function (response) {
alert(response.data);
$scope.Employe.EMPNO = "";
$scope.Employe.ENAME = "";
$scope.Employe.JOB = "";
$scope.Employe.MGR = "";
$scope.Employe.SAL = "";
$scope.Employe.DEPTNO = "";
$scope.GetAllData();
document.getElementById("btnSave").setAttribute("value", "Submit");
document.getElementById("btnSave").style.backgroundColor = "cornflowerblue";
document.getElementById("spn").innerHTML = "Add New Employee";
})
}
}
$scope.GetAllData = function () {
$http({
method: "post",
url: "http://localhost:61289/Employee/GetEmployeeDetails"
}).then(function (response) {
$scope.employees = response.data;
}, function () {
alert("Error Occur");
})
};
$scope.DeleteEmp = function (Emp) {
$http({
method: "post",
url: "http://localhost:61289/Employee/DeleteEmployee",
datatype: "json",
data: JSON.stringify(Emp)
}).then(function (response) {
alert(response.data);
$scope.GetAllData();
})
};
$scope.UpdateEmp = function (Emp) {
document.getElementById("EmpNumber_").value = Emp.EMPNO;
$scope.EmpName = Emp.ENAME;
$scope.EmpJob = Emp.JOB;
$scope.EmpMgrId = Emp.MGR;
$scope.EmpSalary = Emp.SAL;
$scope.EmpNumber = Emp.EMPNO;
$scope.EmpDept = Emp.DEPTNO;
document.getElementById("btnSave").setAttribute("value", "Update");
document.getElementById("btnSave").style.backgroundColor = "Yellow";
document.getElementById("spn").innerHTML = "Update Employee Information";
}
})
You may get Error in the connection String, please make sure that your connection string is Correct
<connectionStrings>
<add name="MYDBEntities" connectionString="metadata=res://*/;provider=System.Data.SqlClient;provider connection string="data source=DESKTOP-HJAK1FL\SQLEXPRESS;initial catalog=MYDB;persist security info=True;user id=sa;Password=1234;MultipleActiveResultSets=True;App=EntityFramework"" providerName="System.Data.EntityClient" />
</connectionStrings>
Index:
@{
ViewBag.Title = "Index";
}
<script src="~/scripts/angular.js"></script>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script src="~/scripts/CrudOperation.js"></script>
<style>
.btn-space {
margin-left: -5%;
background-color: blue;
font-size: large;
}
</style>
<div ng-app="myApp">
<div ng-controller="myController" ng-init="GetAllData()" class="divList">
<p class="divHead">Employee Details</p>
<table cellpadding="12" class="table table-bordered table-hover">
<tr>
<td>
<b>Employee Number</b>
</td>
<td>
<b>Emp Name</b>
</td>
<td>
<b>Job</b>
</td>
<td>
<b>Salary</b>
</td>
<td>
<b>Actions</b>
</td>
</tr>
<tr ng-repeat="Emp in employees">
<td>
{{Emp.EMPNO}}
</td>
<td>
{{Emp.ENAME}}
</td>
<td>
{{Emp.JOB}}
</td>
<td>
{{Emp.SAL}}
</td>
<td>
<input type="button" class="btn btn-warning" value="Update" ng-click="UpdateEmp(Emp)" />
<input type="button" class="btn btn-danger" value="Delete" ng-click="DeleteEmp(Emp)" />
</td>
</tr>
</table>
<div class="form-horizontal" role="form">
<div class="container">
<div class="row">
<h2>
<span id="spn">Add New Employee</span>
</h2>
</div>
<div class="row">
<div class="col-sm-6 col-lg-4">
<div class="form-group">
<label class="col-md-4 control-label">Emp Number:</label>
<div class="col-md-8">
<input type="text" class="form-control" id="inputEmpId" placeholder="Employee Number" ng-model="EmpNumber">
</div>
</div>
</div>
<div class="col-sm-6 col-lg-4">
<div class="form-group">
<label class="col-md-4 control-label">Emp Name:</label>
<div class="col-md-8">
<input type="text" class="form-control" id="inputEmpName" placeholder="Employee Name" ng-model="EmpName">
</div>
</div>
</div>
<div class="col-sm-6 col-lg-4">
<div class="form-group">
<label class="col-md-4 control-label">Job:</label>
<div class="col-md-8">
<input type="text" class="form-control" id="inputJob" placeholder="Employee Designation" ng-model="EmpJob">
</div>
</div>
</div>
<div class="col-sm-6 col-lg-4">
<div class="form-group">
<label class="col-md-4 control-label">Manager ID:</label>
<div class="col-md-8">
<input type="text" class="form-control" id="inputMgrId" placeholder="Id of the Manager" ng-model="EmpMgrId">
</div>
</div>
</div>
<div class="col-sm-6 col-lg-4">
<div class="form-group">
<label class="col-md-4 control-label">Salary :</label>
<div class="col-md-8">
<input type="text" class="form-control" id="inputSalaryId" placeholder="Salary of the Employoee" ng-model="EmpSalary">
</div>
</div>
</div>
<div class="col-sm-6 col-lg-4">
<div class="form-group">
<label class="col-md-4 control-label">Dept Number :</label>
<div class="col-md-8">
<input type="text" class="form-control" id="inputDeptId" placeholder="Department ID of the Employoee" ng-model="EmpDept">
</div>
</div>
</div>
</div>
<div class="row">
<div class="col-sm-6 col-lg-4">
<input type="button" id="btnSave" class="form-control btn-space" value="Submit" ng-click="InsertData()" />
</div>
</div>
</div>
</div>
</div>
@Html.Hidden("EmpNumber_")
</div>
Output:
Click on Ok Button
Design the database as shown below:
Now, Add a new ADO.NET Data Model, From the Model Folder
Click on Add
Click on Next
Click on New Connection
Click on Test Connection. Once the test connection is success
Click on Ok Button
Click on Next Button
Click on Finish Button
Now, we need to Add Angular Js Plugin
Click on Install Button
Once Installed you can see in the script folder
Now, we will Add a EmployeeController and Add the below code
EmployeeController:
public class EmployeeController : Controller
{
// GET: Employee
public ActionResult Index()
{
return View();
}
/// <summary>
/// This method is used to Get the Details of the Employee
/// </summary>
/// <returns>JSONRESULT</returns>
public JsonResult GetEmployeeDetails()
{
using (MYDBEntities Obj = new MYDBEntities())
{
List<EMP> Emp = Obj.EMPs.ToList();
return Json(Emp, JsonRequestBehavior.AllowGet);
}
}
/// <summary>
/// Insert an Employee
/// </summary>
/// <param name="Employe">emp</param>
/// <returns>String</returns>
[HttpPost]
public string InsertEmployee(EMP Employe)
{
if (Employe != null)
{
using (MYDBEntities Obj = new MYDBEntities())
{
Obj.EMPs.Add(Employe);
Obj.SaveChanges();
return "Employee Added Successfully";
}
}
else
{
return "Employee Not Inserted! Try Again";
}
}
/// <summary>
/// This method is used to Delete an Employee
/// </summary>
/// <param name="Employe">emp</param>
/// <returns>String</returns>
public string DeleteEmployee(EMP emp)
{
if (emp != null)
{
using (MYDBEntities Obj = new MYDBEntities())
{
var Emp_ = Obj.Entry(emp);
if (Emp_.State == System.Data.Entity.EntityState.Detached)
{
Obj.EMPs.Attach(emp);
Obj.EMPs.Remove(emp);
}
Obj.SaveChanges();
return "Employee Deleted Successfully";
}
}
else
{
return "Employee Not Deleted! Try Again";
}
}
public string UpdateEmployee(EMP Employe)
{
if (Employe != null)
{
using (MYDBEntities Obj = new MYDBEntities())
{
var Emp_ = Obj.Entry(Employe);
EMP EmpObj = Obj.EMPs.Where(x => x.EMPNO == Employe.EMPNO).FirstOrDefault();
EmpObj.ENAME = Employe.ENAME;
EmpObj.JOB = Employe.JOB;
EmpObj.DEPTNO = Employe.DEPTNO;
EmpObj.MGR = Employe.MGR;
EmpObj.SAL = Employe.SAL;
Obj.SaveChanges();
return "Employee Updated Successfully";
}
}
else
{
return "Employee Not Updated! Try Again";
}
}
}
Javascript Code:
/// <reference path="angular.js" />
var app = angular.module("myApp", []);
app.controller("myController", function ($scope, $http) {
$scope.InsertData = function () {
var Action = document.getElementById("btnSave").getAttribute("value");
if (Action == "Submit") {
$scope.Employe = {};
$scope.Employe.EMPNO = $scope.EmpNumber;
$scope.Employe.ENAME = $scope.EmpName;
$scope.Employe.JOB = $scope.EmpJob;
$scope.Employe.MGR = $scope.EmpMgrId;
$scope.Employe.SAL = $scope.EmpSalary;
$scope.Employe.DEPTNO = $scope.EmpDept;
$http({
method: "post",
url: "http://localhost:61289/Employee/InsertEmployee",
datatype: 'json',
data: JSON.stringify($scope.Employe)
}).then(function (response) {
alert(response.data);
$scope.Employe.EMPNO = "";
$scope.Employe.ENAME = "";
$scope.Employe.JOB = "";
$scope.Employe.MGR = "";
$scope.Employe.SAL = "";
$scope.Employe.DEPTNO = "";
$scope.GetAllData();
})
} else {
debugger;
$scope.Employe = {};
$scope.Employe.ENAME = $scope.EmpName;
$scope.Employe.JOB = $scope.EmpJob;
$scope.Employe.MGR = $scope.EmpMgrId;
$scope.Employe.SAL = $scope.EmpSalary;
$scope.Employe.DEPTNO = $scope.EmpDept;
$scope.Employe.EMPNO = document.getElementById("EmpNumber_").value;
$http({
method: "post",
url: "http://localhost:61289/Employee/UpdateEmployee",
datatype: "json",
data: JSON.stringify($scope.Employe)
}).then(function (response) {
alert(response.data);
$scope.Employe.EMPNO = "";
$scope.Employe.ENAME = "";
$scope.Employe.JOB = "";
$scope.Employe.MGR = "";
$scope.Employe.SAL = "";
$scope.Employe.DEPTNO = "";
$scope.GetAllData();
document.getElementById("btnSave").setAttribute("value", "Submit");
document.getElementById("btnSave").style.backgroundColor = "cornflowerblue";
document.getElementById("spn").innerHTML = "Add New Employee";
})
}
}
$scope.GetAllData = function () {
$http({
method: "post",
url: "http://localhost:61289/Employee/GetEmployeeDetails"
}).then(function (response) {
$scope.employees = response.data;
}, function () {
alert("Error Occur");
})
};
$scope.DeleteEmp = function (Emp) {
$http({
method: "post",
url: "http://localhost:61289/Employee/DeleteEmployee",
datatype: "json",
data: JSON.stringify(Emp)
}).then(function (response) {
alert(response.data);
$scope.GetAllData();
})
};
$scope.UpdateEmp = function (Emp) {
document.getElementById("EmpNumber_").value = Emp.EMPNO;
$scope.EmpName = Emp.ENAME;
$scope.EmpJob = Emp.JOB;
$scope.EmpMgrId = Emp.MGR;
$scope.EmpSalary = Emp.SAL;
$scope.EmpNumber = Emp.EMPNO;
$scope.EmpDept = Emp.DEPTNO;
document.getElementById("btnSave").setAttribute("value", "Update");
document.getElementById("btnSave").style.backgroundColor = "Yellow";
document.getElementById("spn").innerHTML = "Update Employee Information";
}
})
You may get Error in the connection String, please make sure that your connection string is Correct
<connectionStrings>
<add name="MYDBEntities" connectionString="metadata=res://*/;provider=System.Data.SqlClient;provider connection string="data source=DESKTOP-HJAK1FL\SQLEXPRESS;initial catalog=MYDB;persist security info=True;user id=sa;Password=1234;MultipleActiveResultSets=True;App=EntityFramework"" providerName="System.Data.EntityClient" />
</connectionStrings>
Index:
@{
ViewBag.Title = "Index";
}
<script src="~/scripts/angular.js"></script>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script src="~/scripts/CrudOperation.js"></script>
<style>
.btn-space {
margin-left: -5%;
background-color: blue;
font-size: large;
}
</style>
<div ng-app="myApp">
<div ng-controller="myController" ng-init="GetAllData()" class="divList">
<p class="divHead">Employee Details</p>
<table cellpadding="12" class="table table-bordered table-hover">
<tr>
<td>
<b>Employee Number</b>
</td>
<td>
<b>Emp Name</b>
</td>
<td>
<b>Job</b>
</td>
<td>
<b>Salary</b>
</td>
<td>
<b>Actions</b>
</td>
</tr>
<tr ng-repeat="Emp in employees">
<td>
{{Emp.EMPNO}}
</td>
<td>
{{Emp.ENAME}}
</td>
<td>
{{Emp.JOB}}
</td>
<td>
{{Emp.SAL}}
</td>
<td>
<input type="button" class="btn btn-warning" value="Update" ng-click="UpdateEmp(Emp)" />
<input type="button" class="btn btn-danger" value="Delete" ng-click="DeleteEmp(Emp)" />
</td>
</tr>
</table>
<div class="form-horizontal" role="form">
<div class="container">
<div class="row">
<h2>
<span id="spn">Add New Employee</span>
</h2>
</div>
<div class="row">
<div class="col-sm-6 col-lg-4">
<div class="form-group">
<label class="col-md-4 control-label">Emp Number:</label>
<div class="col-md-8">
<input type="text" class="form-control" id="inputEmpId" placeholder="Employee Number" ng-model="EmpNumber">
</div>
</div>
</div>
<div class="col-sm-6 col-lg-4">
<div class="form-group">
<label class="col-md-4 control-label">Emp Name:</label>
<div class="col-md-8">
<input type="text" class="form-control" id="inputEmpName" placeholder="Employee Name" ng-model="EmpName">
</div>
</div>
</div>
<div class="col-sm-6 col-lg-4">
<div class="form-group">
<label class="col-md-4 control-label">Job:</label>
<div class="col-md-8">
<input type="text" class="form-control" id="inputJob" placeholder="Employee Designation" ng-model="EmpJob">
</div>
</div>
</div>
<div class="col-sm-6 col-lg-4">
<div class="form-group">
<label class="col-md-4 control-label">Manager ID:</label>
<div class="col-md-8">
<input type="text" class="form-control" id="inputMgrId" placeholder="Id of the Manager" ng-model="EmpMgrId">
</div>
</div>
</div>
<div class="col-sm-6 col-lg-4">
<div class="form-group">
<label class="col-md-4 control-label">Salary :</label>
<div class="col-md-8">
<input type="text" class="form-control" id="inputSalaryId" placeholder="Salary of the Employoee" ng-model="EmpSalary">
</div>
</div>
</div>
<div class="col-sm-6 col-lg-4">
<div class="form-group">
<label class="col-md-4 control-label">Dept Number :</label>
<div class="col-md-8">
<input type="text" class="form-control" id="inputDeptId" placeholder="Department ID of the Employoee" ng-model="EmpDept">
</div>
</div>
</div>
</div>
<div class="row">
<div class="col-sm-6 col-lg-4">
<input type="button" id="btnSave" class="form-control btn-space" value="Submit" ng-click="InsertData()" />
</div>
</div>
</div>
</div>
</div>
@Html.Hidden("EmpNumber_")
</div>
Output:
No comments:
Post a Comment
Thank you for visiting my blog